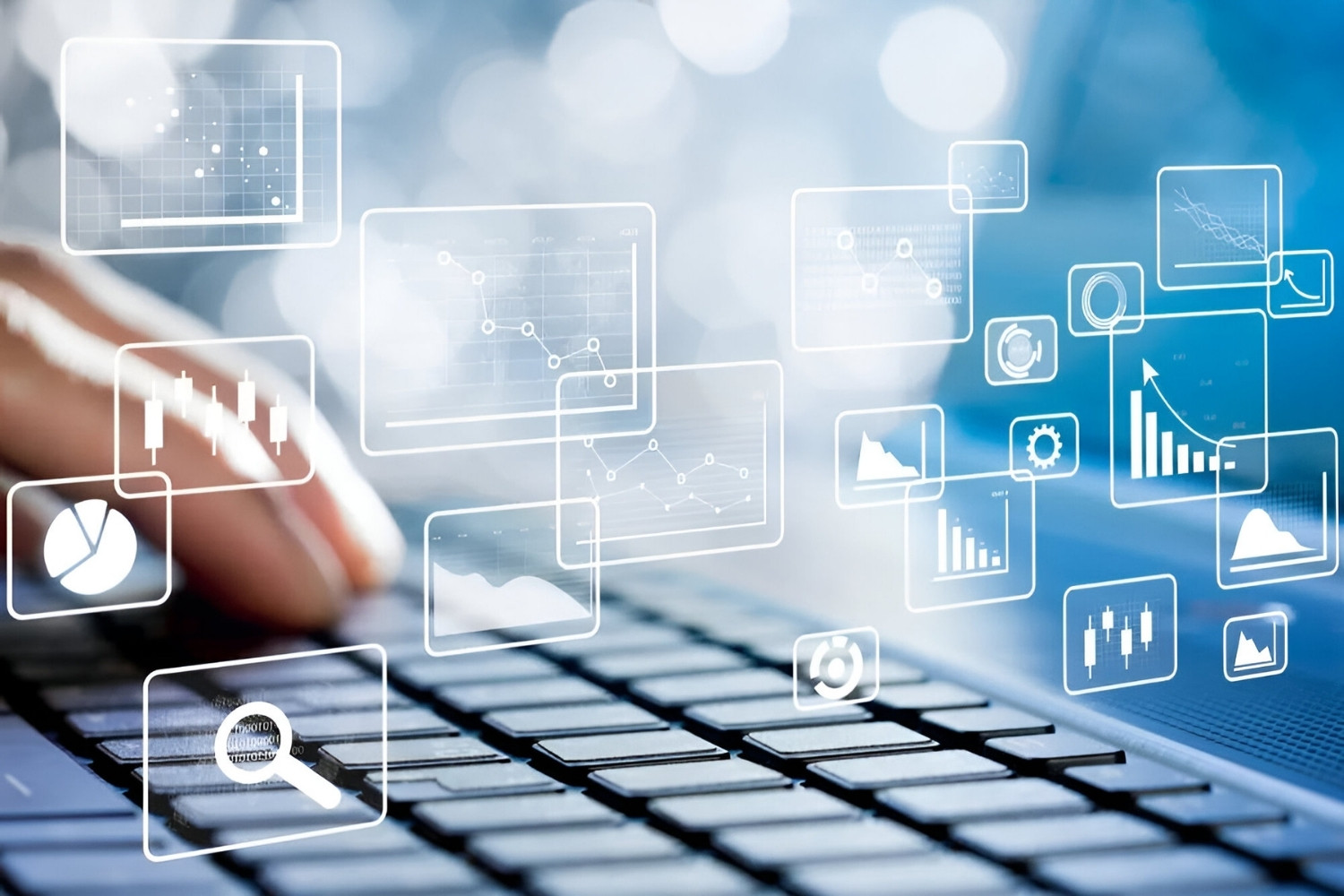
Data types are the building blocks of programming languages. They define the kind of data a variable can hold, whether it's numbers, text, or more complex structures. Understanding data types is crucial for writing efficient and error-free code. But what exactly are data types, and why are they so important? Data types help computers understand how to process different kinds of information. From integers and floating-point numbers to strings and booleans, each type has its own set of rules and uses. In this blog post, we'll explore 32 fascinating facts about data types that will deepen your understanding and appreciation of this fundamental concept in computer science.
What Are Data Types?
Data types are essential in programming and computer science. They define the kind of data that can be stored and manipulated within a program. Understanding data types helps in writing efficient and error-free code.
- 01Integer: Represents whole numbers without fractions. Examples include -1, 0, 42.
- 02Float: Used for numbers with decimal points. Examples are 3.14, -0.001.
- 03Character: Stores single characters like 'a', '1', or '$'.
- 04String: A sequence of characters. For example, "Hello, World!".
- 05Boolean: Represents true or false values. Useful in conditional statements.
Primitive Data Types
Primitive data types are the most basic data types available in programming languages. They are predefined and supported by the language.
- 06Byte: Stores small integers, typically ranging from -128 to 127.
- 07Short: A 16-bit integer, larger than a byte but smaller than an int.
- 08Long: A 64-bit integer, used for very large numbers.
- 09Double: Similar to float but with double precision, allowing for more accurate decimal numbers.
- 10Void: Represents the absence of type. Often used in functions that do not return a value.
Composite Data Types
Composite data types are made up of primitive data types. They allow for more complex data structures.
- 11Array: A collection of elements, all of the same type. For example, an array of integers.
- 12Structure: Groups different data types together. Useful for creating complex data models.
- 13Union: Similar to structures but stores only one of its members at a time.
- 14Enum: A set of named values. Helps in making code more readable and maintainable.
- 15Class: Defines objects in object-oriented programming. Contains attributes and methods.
Abstract Data Types
Abstract data types (ADTs) are not defined by their implementation but by their behavior from the point of view of a user.
- 16List: An ordered collection of elements. Can be implemented using arrays or linked lists.
- 17Stack: Follows Last In, First Out (LIFO) principle. Useful in algorithms like depth-first search.
- 18Queue: Follows First In, First Out (FIFO) principle. Used in scenarios like task scheduling.
- 19Tree: A hierarchical structure with nodes. Commonly used in databases and file systems.
- 20Graph: Consists of nodes and edges. Useful in representing networks.
Specialized Data Types
Some data types are specialized for particular tasks or domains.
- 21Date: Stores dates. Useful in applications that require date manipulation.
- 22Time: Stores time. Often used in scheduling applications.
- 23Timestamp: Combines date and time. Useful in logging events.
- 24Currency: Stores monetary values. Ensures precision in financial applications.
- 25Pointer: Stores memory addresses. Used in languages like C and C++ for dynamic memory allocation.
Data Types in Different Languages
Different programming languages have unique ways of handling data types.
- 26Python: Dynamically typed, meaning variables can change types. Uses types like int, float, str, and list.
- 27Java: Statically typed, meaning variables must be declared with a type. Uses types like int, double, char, and boolean.
- 28JavaScript: Dynamically typed. Common types include number, string, boolean, and object.
- 29C++: Statically typed. Supports primitive types like int, float, and char, as well as complex types like classes and pointers.
- 30SQL: Used for database management. Common types include INT, VARCHAR, DATE, and BOOLEAN.
Importance of Data Types
Understanding data types is crucial for efficient programming and data management.
- 31Memory Management: Different data types require different amounts of memory. Knowing this helps in optimizing memory usage.
- 32Data Integrity: Ensures that the data stored is of the correct type, preventing errors and inconsistencies.
The Final Word on Data Types
Data types are the backbone of programming. They help organize and manage data efficiently. Knowing the difference between integers, floats, strings, and booleans can make coding smoother. Arrays and objects add more complexity but also more power. Null and undefined might seem confusing, but they play crucial roles in handling data that’s not yet available or doesn’t exist. BigInt and symbol are less common but essential for specific tasks. Understanding these types can save time and prevent errors. Whether you’re a beginner or a seasoned coder, mastering data types is a must. They’re not just technical jargon; they’re tools that make your code work. So, keep this guide handy, and you’ll navigate your coding projects with ease. Happy coding!
Was this page helpful?
Our commitment to delivering trustworthy and engaging content is at the heart of what we do. Each fact on our site is contributed by real users like you, bringing a wealth of diverse insights and information. To ensure the highest standards of accuracy and reliability, our dedicated editors meticulously review each submission. This process guarantees that the facts we share are not only fascinating but also credible. Trust in our commitment to quality and authenticity as you explore and learn with us.