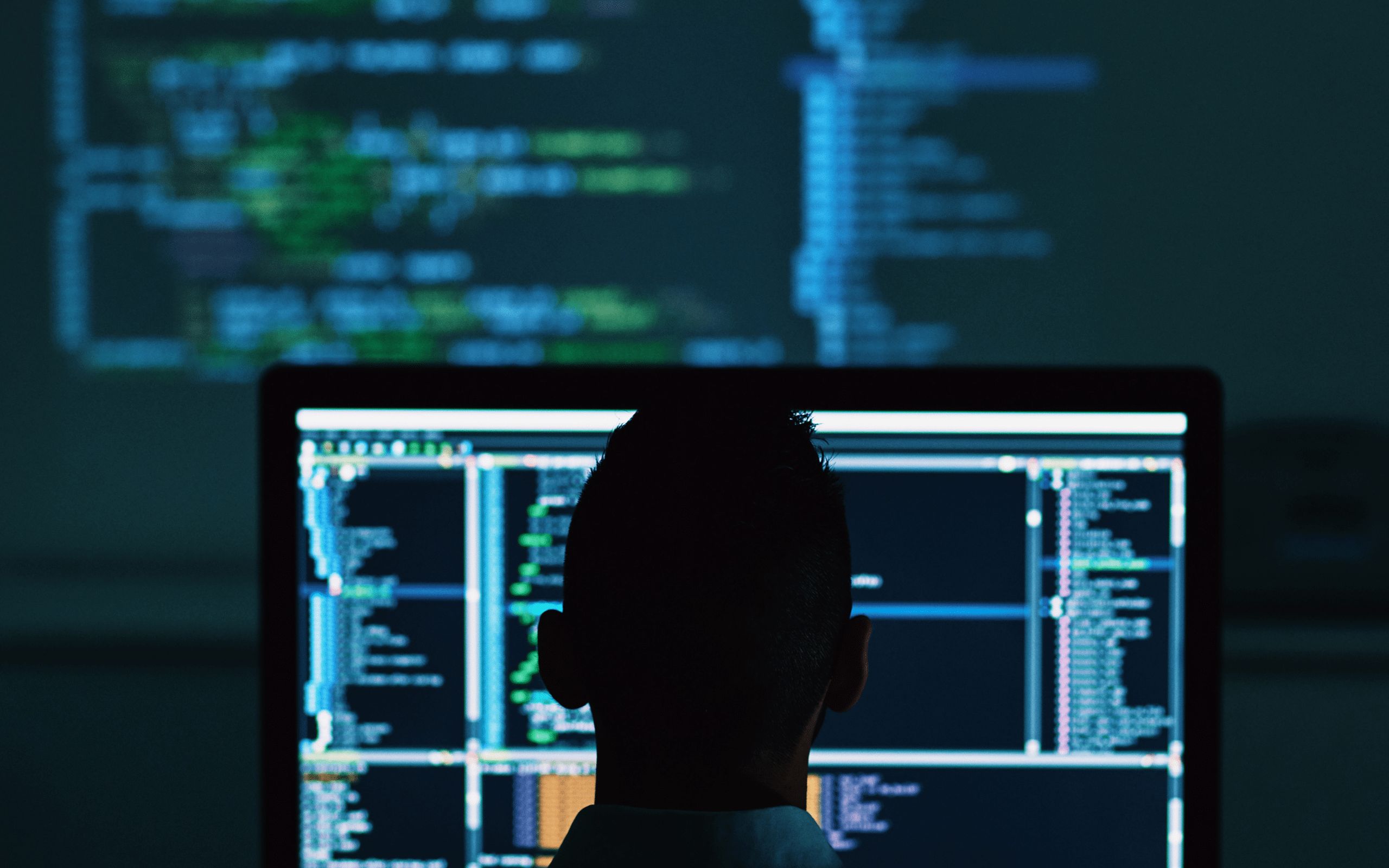
What is the Factory Pattern? The Factory Pattern is a design pattern used in object-oriented programming to create objects without specifying the exact class of the object that will be created. This pattern is particularly useful when the type of object to be created is determined at runtime. By using a factory method, you can encapsulate the object creation process, making your code more modular, scalable, and easier to maintain. This approach also promotes loose coupling between classes, enhancing flexibility. Whether you're a seasoned developer or just starting, understanding the Factory Pattern can significantly improve your coding skills and software design.
What is the Factory Pattern?
The Factory Pattern is a design pattern used in object-oriented programming. It provides a way to create objects without specifying the exact class of the object that will be created. This pattern is particularly useful when the exact type of object isn't known until runtime.
- 01The Factory Pattern is part of the creational design patterns family.
- 02It helps in decoupling the client code from the object creation code.
- 03The pattern is often used in frameworks to allow users to extend and customize the framework without modifying its core code.
- 04It promotes the Single Responsibility Principle by separating the object creation code from the business logic.
- 05The Factory Pattern can be implemented using a static method or an instance method.
Types of Factory Patterns
There are several variations of the Factory Pattern, each with its own unique characteristics and use cases.
- 06Simple Factory: This is the most basic form of the Factory Pattern. It uses a single method to create objects.
- 07Factory Method: This pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created.
- 08Abstract Factory: This pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- 09Static Factory Method: This variation uses a static method to create objects, which can be more convenient but less flexible.
- 10Parameterized Factory: This type allows parameters to be passed to the factory method to influence the type of object created.
Benefits of Using the Factory Pattern
The Factory Pattern offers several advantages that make it a popular choice among developers.
- 11Flexibility: It allows for more flexible and reusable code by decoupling object creation from the main logic.
- 12Maintainability: Changes to the object creation process can be made in one place, making the code easier to maintain.
- 13Testability: It makes unit testing easier by allowing mock objects to be created in place of real objects.
- 14Scalability: New types of objects can be added without modifying existing code.
- 15Consistency: Ensures that objects are created in a consistent manner, reducing the risk of errors.
Real-World Examples of Factory Pattern
The Factory Pattern is widely used in various real-world applications and frameworks.
- 16Java's Calendar Class: The
Calendar.getInstance()
method is a factory method that returns a calendar object. - 17Spring Framework: The Spring Framework uses factory methods to create beans.
- 18Android Development: The
LayoutInflater
class in Android uses the Factory Pattern to create view objects. - 19Database Connections: JDBC uses factory methods to create database connections.
- 20GUI Toolkits: Many GUI toolkits use factory methods to create widgets and controls.
Common Pitfalls and How to Avoid Them
While the Factory Pattern is powerful, it can also lead to some common pitfalls if not used correctly.
- 21Overuse: Overusing the Factory Pattern can lead to overly complex code. Use it only when necessary.
- 22Performance: Factory methods can introduce a slight performance overhead. Be mindful of this in performance-critical applications.
- 23Readability: Excessive use of factory methods can make the code harder to read. Ensure that the factory methods are well-documented.
- 24Testing: While the Factory Pattern can make testing easier, it can also complicate testing if not implemented correctly. Use dependency injection to simplify testing.
- 25Maintenance: If not properly managed, the Factory Pattern can lead to maintenance challenges. Keep the factory methods simple and focused.
Best Practices for Implementing the Factory Pattern
Following best practices can help you get the most out of the Factory Pattern.
- 26Keep it Simple: Start with a simple factory method and evolve it as needed.
- 27Use Interfaces: Define interfaces for the objects being created to promote flexibility and decoupling.
- 28Document: Clearly document the factory methods to make the code easier to understand.
- 29Test: Write unit tests for the factory methods to ensure they work as expected.
- 30Refactor: Regularly refactor the factory methods to keep them clean and maintainable.
Advanced Concepts Related to Factory Pattern
For those looking to dive deeper, there are advanced concepts related to the Factory Pattern worth exploring.
- 31Lazy Initialization: This technique delays the creation of an object until it is needed, which can improve performance.
- 32Singleton Factory: Combines the Singleton and Factory patterns to ensure that only one instance of the factory exists.
- 33Prototype Pattern: Works well with the Factory Pattern by allowing objects to be cloned rather than created from scratch.
- 34Dependency Injection: Often used in conjunction with the Factory Pattern to manage object creation and dependencies.
- 35Service Locator: This pattern can be used to locate and create services, often implemented using factory methods.
Historical Context and Evolution
Understanding the history and evolution of the Factory Pattern can provide valuable insights.
- 36The Factory Pattern was first described in the "Design Patterns: Elements of Reusable Object-Oriented Software" book by the Gang of Four.
- 37It has evolved over time to include various forms and adaptations, such as the Abstract Factory and Factory Method patterns.
- 38The pattern has been widely adopted in modern programming languages and frameworks, making it a staple in software development.
Wrapping Up Factory Patterns
Factory Patterns simplify object creation in programming. They help manage complex code by providing a clear structure. This pattern is widely used in software development, making code more maintainable and scalable. By using Factory Patterns, developers can create objects without specifying the exact class, promoting flexibility and reusability.
Understanding Factory Patterns can boost your coding skills. They are essential for creating efficient, clean, and organized code. Whether you're a beginner or an experienced developer, mastering this pattern can significantly improve your programming practices.
Incorporating Factory Patterns into your projects can lead to better performance and easier maintenance. They are a fundamental concept in object-oriented programming, offering a robust solution for managing object creation. So, dive into Factory Patterns and see how they can transform your coding experience. Happy coding!
Was this page helpful?
Our commitment to delivering trustworthy and engaging content is at the heart of what we do. Each fact on our site is contributed by real users like you, bringing a wealth of diverse insights and information. To ensure the highest standards of accuracy and reliability, our dedicated editors meticulously review each submission. This process guarantees that the facts we share are not only fascinating but also credible. Trust in our commitment to quality and authenticity as you explore and learn with us.