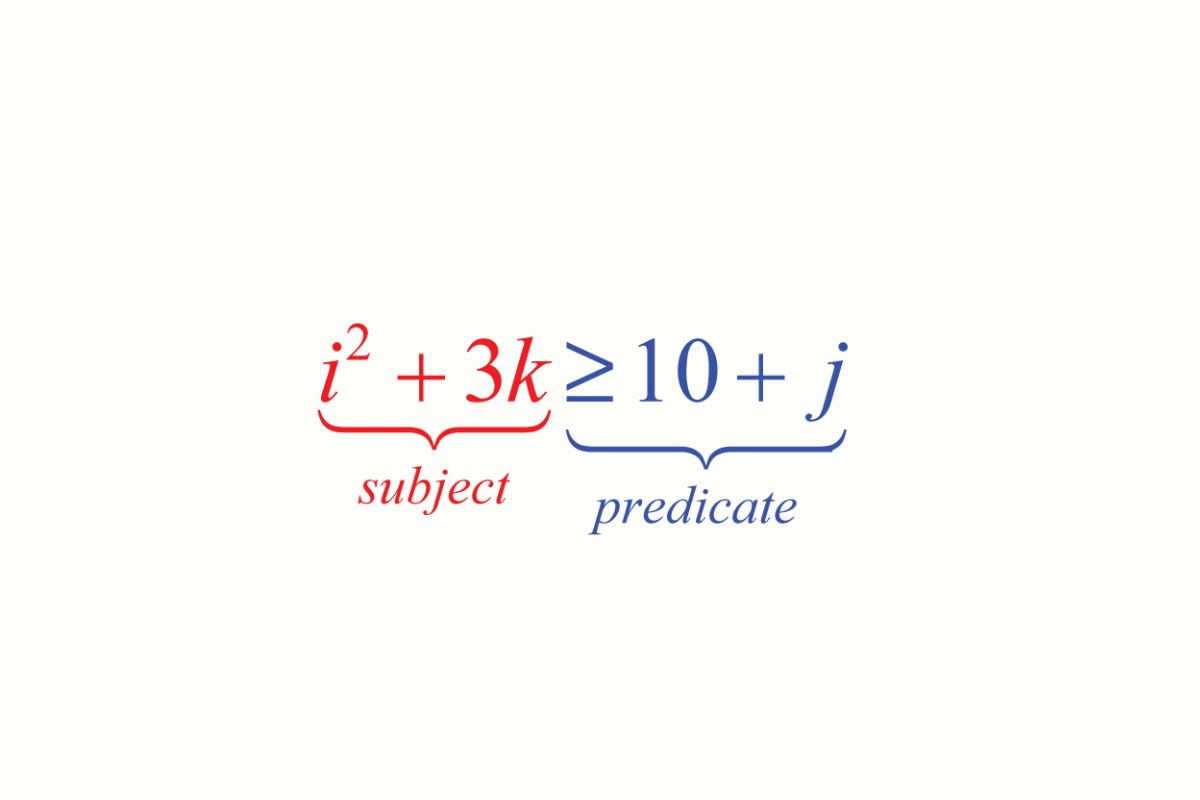
What are predicate methods? Predicate methods are special functions in programming that return a boolean value, either true or false. They help determine if a certain condition or property holds for a given object or data set. These methods are often used in filtering, searching, and validating data. For example, in Ruby, predicate methods typically end with a question mark, like empty?
or even?
. In Python, they might be named is_empty
or is_even
. Understanding predicate methods can make your code more readable and efficient. Ready to dive into the world of predicate methods? Let's explore 29 fascinating facts about them!
What Are Predicate Methods?
Predicate methods are functions or procedures in programming that return a boolean value: true or false. They are often used to make decisions in code, such as checking conditions or validating data. Here are some intriguing facts about predicate methods.
- 01
Boolean Return Type: Predicate methods always return a boolean value, either true or false. This makes them perfect for conditional statements.
- 02
Common in Functional Programming: Languages like Haskell and Lisp heavily use predicate methods due to their functional nature.
- 03
Naming Conventions: In many programming languages, predicate methods often start with "is" or "has" to indicate they return a boolean. For example,
isEmpty()
orhasChildren()
. - 04
Used in Filtering: Predicate methods are frequently used in filtering data structures like lists or arrays. For instance, filtering out even numbers from a list.
- 05
Simplify Code: By encapsulating conditions within predicate methods, code readability and maintainability improve significantly.
How Predicate Methods Work
Understanding how predicate methods function can help in writing more efficient code. Here are some facts about their workings.
- 06
Conditional Checks: They perform conditional checks within their body and return true or false based on the outcome.
- 07
Short-Circuit Evaluation: In languages like JavaScript, predicate methods can benefit from short-circuit evaluation, where the evaluation stops as soon as the result is determined.
- 08
Lambda Expressions: In modern programming languages, predicate methods can be implemented using lambda expressions, making the code more concise.
- 09
Higher-Order Functions: Predicate methods can be passed as arguments to higher-order functions, which are functions that take other functions as parameters.
- 10
Error Handling: Proper error handling within predicate methods ensures that they return a boolean value even when exceptions occur.
Examples of Predicate Methods
Examples can make the concept of predicate methods clearer. Here are some common examples.
- 11
isEmpty(): Checks if a data structure like a list or a string is empty.
- 12
isPrime(): Determines if a number is a prime number.
- 13
hasPermission(): Checks if a user has the necessary permissions to perform an action.
- 14
isValidEmail(): Validates if a string is in the correct email format.
- 15
isPalindrome(): Checks if a string reads the same forwards and backwards.
Benefits of Using Predicate Methods
Predicate methods offer several advantages in programming. Here are some benefits.
- 16
Code Reusability: They promote code reusability by encapsulating common conditions in a single method.
- 17
Improved Readability: Code becomes more readable and understandable when conditions are abstracted into predicate methods.
- 18
Easier Testing: Predicate methods can be easily tested in isolation, ensuring that the conditions they check are correct.
- 19
Modularity: They contribute to the modularity of code, making it easier to manage and maintain.
- 20
Consistency: Using predicate methods ensures consistency in how conditions are checked throughout the codebase.
Challenges with Predicate Methods
Despite their benefits, predicate methods can also present some challenges. Here are a few.
- 21
Performance Overhead: Overuse of predicate methods can lead to performance overhead, especially if they involve complex calculations.
- 22
Debugging Difficulty: Debugging issues related to predicate methods can be tricky, particularly when they are used extensively.
- 23
Dependency Management: Managing dependencies within predicate methods can become complicated, especially in large codebases.
- 24
State Management: Predicate methods that rely on external state can lead to unpredictable behavior if the state changes unexpectedly.
- 25
Complexity: Writing predicate methods for complex conditions can be challenging and may require careful planning.
Best Practices for Predicate Methods
Following best practices can help in effectively using predicate methods. Here are some tips.
- 26
Keep Them Simple: Ensure that predicate methods are simple and focused on a single condition.
- 27
Use Descriptive Names: Use descriptive names that clearly indicate what the method checks.
- 28
Avoid Side Effects: Predicate methods should not have side effects; they should only return a boolean value.
- 29
Document Them: Properly document predicate methods to explain what conditions they check and why.
Final Thoughts on Predicate Methods
Predicate methods are essential tools in programming, helping to streamline code and make it more readable. They allow developers to create functions that return true or false based on specific conditions. This makes code easier to debug and maintain. Understanding how to use predicate methods effectively can save time and reduce errors. They are widely used in various programming languages, including Ruby, Python, and JavaScript. By mastering predicate methods, developers can write cleaner, more efficient code. This not only improves the quality of the software but also enhances the overall development process. So, if you're looking to improve your coding skills, focusing on predicate methods is a great place to start. They might seem simple, but their impact on your code can be profound. Happy coding!
Was this page helpful?
Our commitment to delivering trustworthy and engaging content is at the heart of what we do. Each fact on our site is contributed by real users like you, bringing a wealth of diverse insights and information. To ensure the highest standards of accuracy and reliability, our dedicated editors meticulously review each submission. This process guarantees that the facts we share are not only fascinating but also credible. Trust in our commitment to quality and authenticity as you explore and learn with us.