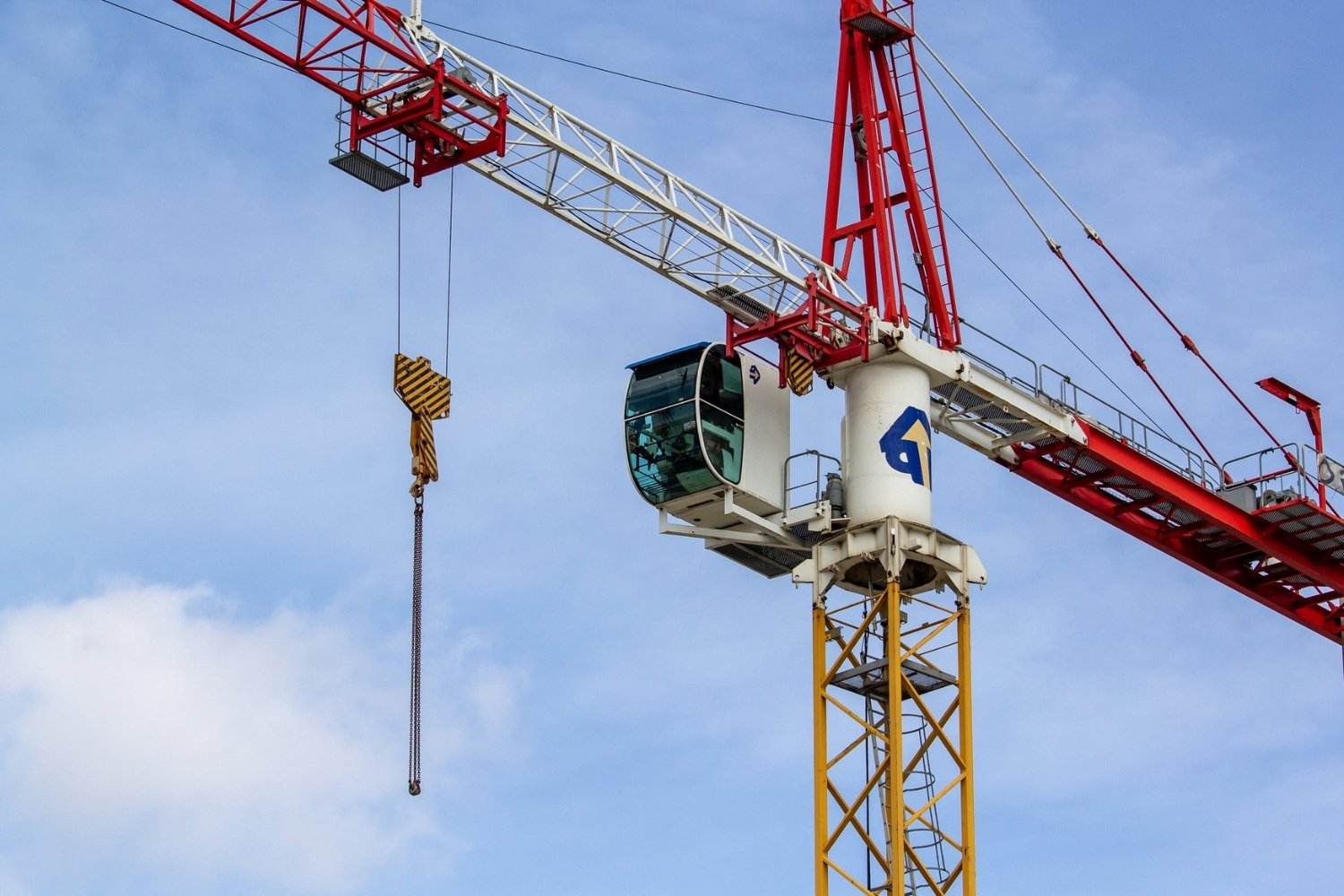
What is hoisting in JavaScript? Hoisting in JavaScript is a behavior where variable and function declarations are moved to the top of their containing scope during the compile phase. This means you can use functions and variables before declaring them in your code. However, only the declarations are hoisted, not the initializations. For example, if you declare a variable but assign it a value later, the declaration is hoisted, but the assignment remains where it is. This can lead to unexpected results if you're not careful. Understanding hoisting helps avoid bugs and write cleaner, more predictable code.
What is Hoisting?
Hoisting is a concept in JavaScript that allows variables and functions to be used before they are declared. This can be confusing for beginners but is a fundamental aspect of how JavaScript works.
- 01Hoisting moves declarations to the top of their scope, not the actual assignments.
- 02Variables declared with
var
are hoisted, but their initializations are not. - 03Functions declared using function declarations are fully hoisted, including their definitions.
- 04Function expressions are not hoisted; only the variable declaration is.
- 05Let and const declarations are hoisted but remain in a "temporal dead zone" until they are initialized.
How Hoisting Works
Understanding how hoisting works can help you write better and more predictable code. Here are some key points to consider.
- 06JavaScript engine processes declarations first, then executes the code.
- 07Function declarations are hoisted before variable declarations.
- 08Temporal dead zone is the period between entering the scope and the actual declaration.
- 09Using a variable before declaring it with
let
orconst
will throw a ReferenceError. - 10Hoisting does not occur in block scopes created by
let
andconst
.
Examples of Hoisting
Examples can make the concept of hoisting clearer. Let's look at some common scenarios.
- 11
Example 1: Using a
var
variable before its declaration.
javascript
console.log(x); // undefined
var x = 5; - 12
Example 2: Using a
let
variable before its declaration.
javascript
console.log(y); // ReferenceError
let y = 5; - 13
Example 3: Function declaration hoisting.
javascript
greet(); // "Hello"
function greet() {
console.log("Hello");
} - 14
Example 4: Function expression hoisting.
javascript
greet(); // TypeError: greet is not a function
var greet = function() {
console.log("Hello");
};
Common Pitfalls
Hoisting can lead to some common pitfalls if not understood properly. Here are a few to watch out for.
- 15Assuming
let
andconst
behave likevar
can cause errors. - 16Misunderstanding the temporal dead zone can lead to unexpected ReferenceErrors.
- 17Overusing
var
can lead to bugs due to its function-scoped nature. - 18Forgetting that function expressions are not hoisted can cause runtime errors.
- 19Confusing function declarations with function expressions can lead to logical errors.
Best Practices
Following best practices can help you avoid issues related to hoisting. Here are some tips.
- 20Always declare variables at the top of their scope.
- 21Use
let
andconst
instead ofvar
to avoid hoisting-related issues. - 22Declare functions before calling them to make the code more readable.
- 23Avoid using the same variable name in nested scopes.
- 24Understand the difference between function declarations and expressions.
Advanced Hoisting Concepts
For those who want to dive deeper, here are some advanced concepts related to hoisting.
- 25Class declarations are hoisted but not initialized.
- 26Arrow functions behave like function expressions and are not hoisted.
- 27Modules in JavaScript have their own scope and hoisting rules.
- 28Async functions and
await
can introduce new hoisting behaviors. - 29Generators also follow hoisting rules but have unique execution contexts.
- 30Closures can capture hoisted variables, leading to unexpected behaviors.
Final Thoughts on Hoisting
Hoisting, a fundamental concept in JavaScript, can seem tricky at first. Understanding that variable and function declarations are moved to the top of their scope during compilation helps in writing cleaner code. Remember, var declarations are hoisted and initialized with undefined
, while let and const are hoisted but not initialized. This can lead to the "Temporal Dead Zone" if not handled properly.
Function declarations are fully hoisted, making them accessible anywhere in their scope. However, function expressions behave like variables, only becoming available after their definition. Knowing these nuances can prevent bugs and improve code readability.
Mastering hoisting empowers developers to write more efficient and predictable JavaScript. Keep experimenting and practicing to solidify your understanding. Happy coding!
Was this page helpful?
Our commitment to delivering trustworthy and engaging content is at the heart of what we do. Each fact on our site is contributed by real users like you, bringing a wealth of diverse insights and information. To ensure the highest standards of accuracy and reliability, our dedicated editors meticulously review each submission. This process guarantees that the facts we share are not only fascinating but also credible. Trust in our commitment to quality and authenticity as you explore and learn with us.